How to convert Markdown to HTML
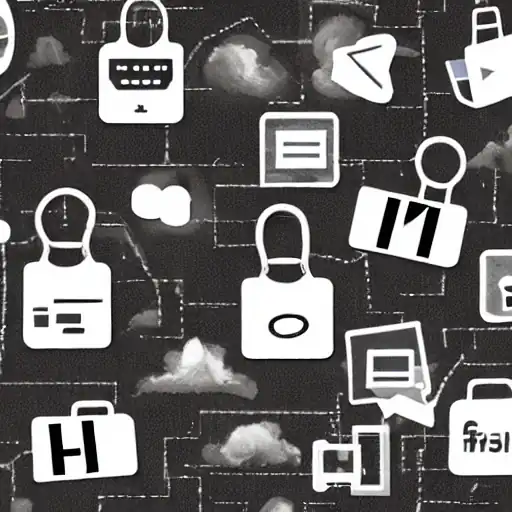
Overview
With remark, you can convert Markdown to HTML. You can also turn HTML into markdown.
Markdown to HTML
Conversion is done with a combination of several plug-ins.
rehype-parse => rehype-remark => remark-stringify
remark-parse: convert from markdown to mdast
remark-rehype: convert from mdast to hast
rehype-stringify: convert from hast to HTML
mdst is Markdown Abstract Syntax Tree.
hast is Hypertext Abstract Syntax Tree.
Make intermediate conversions easier by converting to an abstract syntax tree.
For example, syntax highlighting.
Install packages.
npm i unified remark-parse remark-rehype rehype-stringify
As mentioned earlier, arrange the plugins.
unified is a processor that handles a series of processes.
import { unified } from "unified";
import remarkParse from "remark-parse";
import remarkRehype from "remark-rehype";
import rehypeStringify from "rehype-stringify";
async function markdownToHtml(input: string) {
const processor = unified()
.use(remarkParse)
.use(remarkRehype)
.use(rehypeStringify);
const vfile = await processor.process(input);
const html = vfile.toString();
return html;
}
For example, pass the following markdown.
# Markdown to HTML
this is **test**.
Here is the result.
<h1>Markdown to HTML</h1>
<p>this is <strong>test</strong>.</p>
HTML to Markdown
You can convert markdown to HTML by reversing the flow.
The plougins used are as follows.
rehype-parse => rehype-remark => remark-stringify
rehype-parse: convert from HTML to hast
rehype-remark: convert from hast to mdast
remark-stringify: convert from mdast to markdown
import { unified } from "unified";
import rehypeParse from "rehype-parse";
import rehypeRemark from "rehype-remark";
import remarkStringify from "remark-stringify";
async function htmlToMarkdown(input: string) {
const processor = unified()
.use(rehypeParse)
.use(rehypeRemark)
.use(remarkStringify);
const vfile = await processor.process(input);
const markdown = vfile.toString();
return markdown;
}
For example , pass the following HTML.
<h1>Markdown to HTML</h1>
<p>this is <strong>test</strong>.</p>
Here is the result.
# Markdown to HTML
this is **test**.
Reference
Remark で広げる Markdown の世界
unified を使う前準備
Next.js の静的サイトに Prism を使ってシンタックスハイライトを当てる