How to convert images to WebP
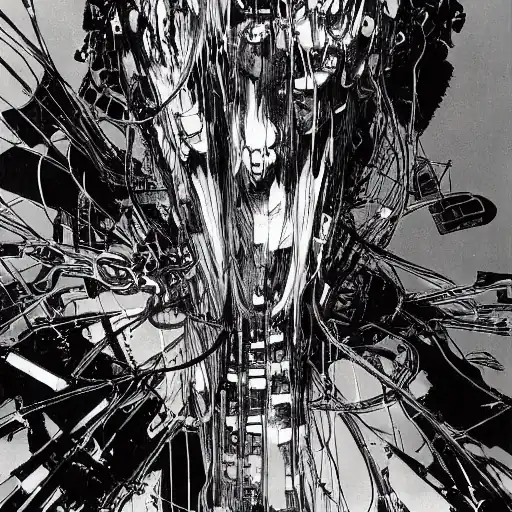
Overview
Converting images to WebP. We can reduce image file size with sacrificing quality by using the WebP format.
What is WebP?
It's the image format developed by Google. It can reduce image file size while maintaining the quality of the image. So, it reduces image loading time.
WebP lossless images are 26% smaller in size compared to PNGs. WebP lossy images are 25-34% smaller than comparable JPEG images at equivalent SSIM quality index.
-- An image format for the Web
WebP is supported by major browsers such as Chrome, Safari, Firefox, Edge, and Opera.
You can check here.
You can also display WebP only on compatible browsers.
<picture>
<source srcset="image.webp" type="image/webp" />
<img src="image.png" />
<picture></picture
></picture>
Conversion service on the web
It is useful for converting images one by one while checking their quality.
For example, you can convert with this google-provided web app.
squoosh
Here you can use sample images from the site to see the effect without having to prepare images. You can try it out.
Conversion library
Some popular libraries available for nodejs include
- libSquoosh
- imagemin-webp
- sharp
I will show you how to use imagemin-webp.
npm i imagemin imagemin-webp
Conver jpg and png under "images" folder to WebP.
Converted files are saved in "build/images"
import imagemin from "imagemin";
import imageminWebp from "imagemin-webp";
async function conv() {
await imagemin(["images/*.{jpg,png}"], {
destination: "build/images",
plugins: [imageminWebp({ quality: 50 })],
});
}
Conversion of images used in the blog
This image was converted to WebP for testing.
The quality is not particularly uncomfortable.
Before conversion: 600KB png.
After conversion: 65KB WebP.
The file size has been reduced by 89%!
Rating at page insights
I tried to see how converting the images on this website to webp would change the page insights rating. Only 4 articles at this time and 4 images to be converted.
The file sizes of the images used are as follows.
format | before | after | change |
---|---|---|---|
JPG | 153 | 9 | 94%down |
PNG | 600 | 65 | 89%down |
PNG | 474 | 22 | 95%down |
PNG | 590 | 47 | 92%down |
mobile performance comparison
before converting
after converting
Largest Contentful Paint has been greatly improved. The rating went up from 78 to 91 just by changing to WebP.
desktop performance comparison
before converting
after converting
Largest Contentful Paint is also faster by replacing with WebP.
I don't know why Cumulative Layout Shift has gone up.
I haven't changed anything other than the image format.
I suspect that it is probably a blur in the Page Insights evaluation.
The effect of reducing the file size in WebP is mainly to shortern the Largest Contentful Paint,
so i will not pursue it here.
Next.js and Images
If you're using Next.js, using Next/Image can improve image-related issues and improve website display performance. It automatically convert image format to WebP, so you don't need to prepare WebP images in advance.