Creating blog using Next.js
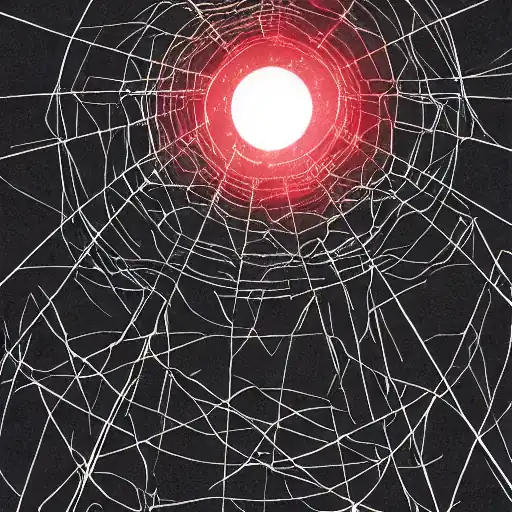
Create Blog
Create a blog base from the next.js sample. I can create post articles by writing md files. The md files are converted to HTML in advance in getStaticProps called at build time.
npx create-next-app --example blog-starter blog-starter-app
cd blog-starter-app
npm run dev
MD file conversion flow
Obtain the path of the md file by "fs.readdirSync(postsDirectory)".
Acquire the data in the md file by gray-matter and separate the content s from the data. Specifically, it acquires the title, author's image address, etc.
Convert the md file to HTML with remark.
Typography
Articles created by converting markdown have almost no css applied to them, so it will be difficult to read as it is. Instead of editing "components/markdown-styles.module.css" directly to style it, you can easily style it using @tailwindcss/typography.
npm install -D @tailwindcss/typography
Add the plugin to tailwind.config.js file.
module.exports = {
content: ['./components/**/*.tsx', './pages/**/*.tsx'],
theme: {
// ...
},
},
plugins: [
require('@tailwindcss/typography'),
],
}
Usage is simple. Just add "prose" to the class where you need it. I added it to the [slug].tsx article tag.
<article className="mb-32 prose"></article>
Syntax highlight
When placing code blocks, syntax highlighting makes them easier to read. Prism.js provides an easy solution. remark-prism is Prism.js that can be used with remark.
npm install remark-prism
The usage is as follows.
import { remark } from "remark";
import html from "remark-html";
import prism from "remark-prism";
export default async function markdownToHtml(markdown: string) {
const result = await remark()
.use(html, { sanitize: false })
.use(prism)
.process(markdown);
return result.toString();
}
Styles are applied when importing a theme. There are several themes available, so choose the one you like best.
/** _app.tsx */
import "prismjs/themes/prism-coy.css";
// import 'prismjs/themes/prism-dark.css'
// import 'prismjs/themes/prism-funky.css'
// import 'prismjs/themes/prism-okaidia.css'
// import 'prismjs/themes/prism-solarizedlight.css'
// import 'prismjs/themes/prism-tomorrow.css'
// import 'prismjs/themes/prism-twilight.css'
// import 'prismjs/themes/prism.css'
After that, Iadjusted the style in my case.
/** index.css */
div.remark-highlight pre {
@apply text-sm;
}
References
https://www.npmjs.com/package/gray-matter
https://www.npmjs.com/package/remark
https://nextjs.org/docs/basic-features/data-fetching/get-static-props
https://reffect.co.jp/react/nextjs-markdown-blog